-
Notifications
You must be signed in to change notification settings - Fork 12
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
13 changed files
with
931 additions
and
1 deletion.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,24 @@ | ||
name: build | ||
|
||
on: | ||
push: | ||
branches: [ master ] | ||
pull_request: | ||
branches: [ master ] | ||
|
||
jobs: | ||
test: | ||
strategy: | ||
matrix: | ||
go: [ '1.13.x', '1.14.x', '1.15.x', '1.16.x' ] | ||
runs-on: ubuntu-latest | ||
steps: | ||
- uses: actions/checkout@master | ||
- name: Set up Go | ||
uses: actions/setup-go@v1 | ||
with: | ||
go-version: ${{ matrix.go }} | ||
- name: test | ||
run: go test -coverprofile=coverage.txt -covermode=atomic | ||
- name: coverage | ||
run: bash <(curl -s https://codecov.io/bash) |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,8 @@ | ||
**Describe the PR** | ||
e.g. add cool parser. | ||
|
||
**Relation issue** | ||
e.g. https://github.com/swaggo/gin-swagger/pull/123/files | ||
|
||
**Additional context** | ||
Add any other context about the problem here. |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,2 +1,75 @@ | ||
# fiber-swagger | ||
echo middleware to automatically generate RESTful API documentation with Swagger 2.0. | ||
fiber middleware to automatically generate RESTful API documentation with Swagger 2.0. | ||
|
||
[](https://github.com/features/actions) | ||
[](https://codecov.io/gh/swaggo/fiber-swagger) | ||
[](https://goreportcard.com/report/github.com/swaggo/fiber-swagger) | ||
[](https://github.com/swaggo/echo-swagger/releases) | ||
|
||
|
||
## Usage | ||
|
||
### Start using it | ||
1. Add comments to your API source code, [See Declarative Comments Format](https://github.com/swaggo/swag#declarative-comments-format). | ||
2. Download [Swag](https://github.com/swaggo/swag) for Go by using: | ||
```sh | ||
$ go get github.com/swaggo/swag/cmd/swag | ||
``` | ||
3. Run the [Swag](https://github.com/swaggo/swag) in your Go project root folder which contains `main.go` file, [Swag](https://github.com/swaggo/swag) will parse comments and generate required files(`docs` folder and `docs/doc.go`). | ||
```sh_ "github.com/swaggo/echo-swagger/v2/example/docs" | ||
$ swag init | ||
``` | ||
4. Download [fiber-swagger](https://github.com/swaggo/fiber-swagger) by using: | ||
```sh | ||
$ go get -u github.com/swaggo/fiber-swagger | ||
``` | ||
|
||
And import following in your code: | ||
```go | ||
import "github.com/swaggo/fiber-swagger" // fiber-swagger middleware | ||
``` | ||
|
||
### Canonical example: | ||
|
||
```go | ||
package main | ||
|
||
import ( | ||
"log" | ||
|
||
"github.com/gofiber/fiber/v2" | ||
"github.com/swaggo/fiber-swagger" | ||
|
||
_ "github.com/swaggo/fiber-swagger/example/docs" // docs is generated by Swag CLI, you have to import it. | ||
) | ||
|
||
// @title Swagger Example API | ||
// @version 1.0 | ||
// @description This is a sample server Petstore server. | ||
// @termsOfService http://swagger.io/terms/ | ||
|
||
// @contact.name API Support | ||
// @contact.url http://www.swagger.io/support | ||
// @contact.email [email protected] | ||
|
||
// @license.name Apache 2.0 | ||
// @license.url http://www.apache.org/licenses/LICENSE-2.0.html | ||
|
||
// @host petstore.swagger.io | ||
// @BasePath /v2 | ||
func main() { | ||
app := fiber.New() | ||
|
||
app.Get("/swagger/*", fiberSwagger.WrapHandler) | ||
|
||
err := app.Listen(":1323") | ||
if err != nil { | ||
log.Fatalf("fiber.Listen failed %s",err) | ||
} | ||
} | ||
|
||
``` | ||
|
||
5. Run it, and browser to http://localhost:1323/swagger/index.html, you can see Swagger 2.0 Api documents. | ||
|
||
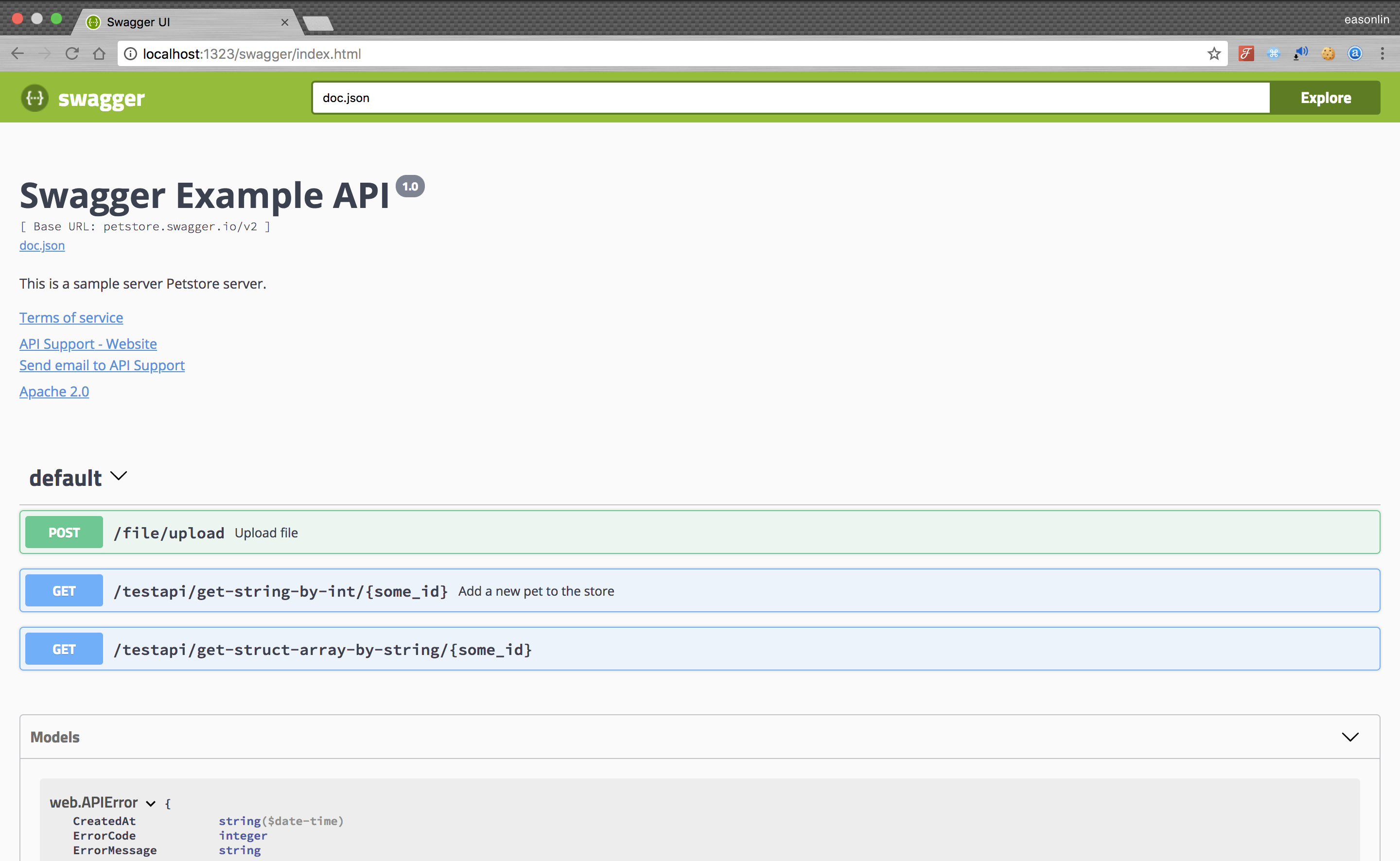 |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,89 @@ | ||
// Package docs GENERATED BY THE COMMAND ABOVE; DO NOT EDIT | ||
// This file was generated by swaggo/swag | ||
package docs | ||
|
||
import ( | ||
"bytes" | ||
"encoding/json" | ||
"strings" | ||
"text/template" | ||
|
||
"github.com/swaggo/swag" | ||
) | ||
|
||
var doc = `{ | ||
"schemes": {{ marshal .Schemes }}, | ||
"swagger": "2.0", | ||
"info": { | ||
"description": "{{escape .Description}}", | ||
"title": "{{.Title}}", | ||
"termsOfService": "http://swagger.io/terms/", | ||
"contact": { | ||
"name": "API Support", | ||
"url": "http://www.swagger.io/support", | ||
"email": "[email protected]" | ||
}, | ||
"license": { | ||
"name": "Apache 2.0", | ||
"url": "http://www.apache.org/licenses/LICENSE-2.0.html" | ||
}, | ||
"version": "{{.Version}}" | ||
}, | ||
"host": "{{.Host}}", | ||
"basePath": "{{.BasePath}}", | ||
"paths": {} | ||
}` | ||
|
||
type swaggerInfo struct { | ||
Version string | ||
Host string | ||
BasePath string | ||
Schemes []string | ||
Title string | ||
Description string | ||
} | ||
|
||
// SwaggerInfo holds exported Swagger Info so clients can modify it | ||
var SwaggerInfo = swaggerInfo{ | ||
Version: "1.0", | ||
Host: "petstore.swagger.io", | ||
BasePath: "/v2", | ||
Schemes: []string{}, | ||
Title: "Swagger Example API", | ||
Description: "This is a sample server Petstore server.", | ||
} | ||
|
||
type s struct{} | ||
|
||
func (s *s) ReadDoc() string { | ||
sInfo := SwaggerInfo | ||
sInfo.Description = strings.Replace(sInfo.Description, "\n", "\\n", -1) | ||
|
||
t, err := template.New("swagger_info").Funcs(template.FuncMap{ | ||
"marshal": func(v interface{}) string { | ||
a, _ := json.Marshal(v) | ||
return string(a) | ||
}, | ||
"escape": func(v interface{}) string { | ||
// escape tabs | ||
str := strings.Replace(v.(string), "\t", "\\t", -1) | ||
// replace " with \", and if that results in \\", replace that with \\\" | ||
str = strings.Replace(str, "\"", "\\\"", -1) | ||
return strings.Replace(str, "\\\\\"", "\\\\\\\"", -1) | ||
}, | ||
}).Parse(doc) | ||
if err != nil { | ||
return doc | ||
} | ||
|
||
var tpl bytes.Buffer | ||
if err := t.Execute(&tpl, sInfo); err != nil { | ||
return doc | ||
} | ||
|
||
return tpl.String() | ||
} | ||
|
||
func init() { | ||
swag.Register(swag.Name, &s{}) | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,21 @@ | ||
{ | ||
"swagger": "2.0", | ||
"info": { | ||
"description": "This is a sample server Petstore server.", | ||
"title": "Swagger Example API", | ||
"termsOfService": "http://swagger.io/terms/", | ||
"contact": { | ||
"name": "API Support", | ||
"url": "http://www.swagger.io/support", | ||
"email": "[email protected]" | ||
}, | ||
"license": { | ||
"name": "Apache 2.0", | ||
"url": "http://www.apache.org/licenses/LICENSE-2.0.html" | ||
}, | ||
"version": "1.0" | ||
}, | ||
"host": "petstore.swagger.io", | ||
"basePath": "/v2", | ||
"paths": {} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,16 @@ | ||
basePath: /v2 | ||
host: petstore.swagger.io | ||
info: | ||
contact: | ||
email: [email protected] | ||
name: API Support | ||
url: http://www.swagger.io/support | ||
description: This is a sample server Petstore server. | ||
license: | ||
name: Apache 2.0 | ||
url: http://www.apache.org/licenses/LICENSE-2.0.html | ||
termsOfService: http://swagger.io/terms/ | ||
title: Swagger Example API | ||
version: "1.0" | ||
paths: {} | ||
swagger: "2.0" |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,35 @@ | ||
package main | ||
|
||
import ( | ||
"log" | ||
|
||
"github.com/gofiber/fiber/v2" | ||
"github.com/swaggo/fiber-swagger" | ||
|
||
_ "github.com/swaggo/fiber-swagger/example/docs" // docs is generated by Swag CLI, you have to import it. | ||
) | ||
|
||
// @title Swagger Example API | ||
// @version 1.0 | ||
// @description This is a sample server Petstore server. | ||
// @termsOfService http://swagger.io/terms/ | ||
|
||
// @contact.name API Support | ||
// @contact.url http://www.swagger.io/support | ||
// @contact.email [email protected] | ||
|
||
// @license.name Apache 2.0 | ||
// @license.url http://www.apache.org/licenses/LICENSE-2.0.html | ||
|
||
// @host petstore.swagger.io | ||
// @BasePath /v2 | ||
func main() { | ||
app := fiber.New() | ||
|
||
app.Get("/swagger/*", fiberSwagger.WrapHandler) | ||
|
||
err := app.Listen(":1323") | ||
if err != nil { | ||
log.Fatalf("fiber.Listen failed %s", err) | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,11 @@ | ||
module github.com/swaggo/fiber-swagger | ||
|
||
go 1.13 | ||
|
||
require ( | ||
github.com/gofiber/fiber/v2 v2.20.2 | ||
github.com/stretchr/testify v1.7.0 | ||
github.com/swaggo/files v0.0.0-20210815190702-a29dd2bc99b2 | ||
github.com/swaggo/swag v1.7.3 | ||
github.com/valyala/fasthttp v1.30.0 | ||
) |
Oops, something went wrong.