-
-
Notifications
You must be signed in to change notification settings - Fork 406
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
14 changed files
with
320 additions
and
0 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,17 @@ | ||
# Bitcoin Chart | ||
This example creates a CodeInterpreterSession and generates a response to plot the bitcoin chart for year 2023: | ||
|
||
```python | ||
from codeinterpreterapi import CodeInterpreterSession | ||
|
||
with CodeInterpreterSession() as session: | ||
response = session.generate_response("Plot the bitcoin chart for year 2023") | ||
|
||
print(response.content) | ||
response.files[0].show_image() # Show the chart image | ||
``` | ||
|
||
The session handles executing the python code to generate the chart in the sandboxed environment. The response contains the chart image that can be displayed. | ||
|
||
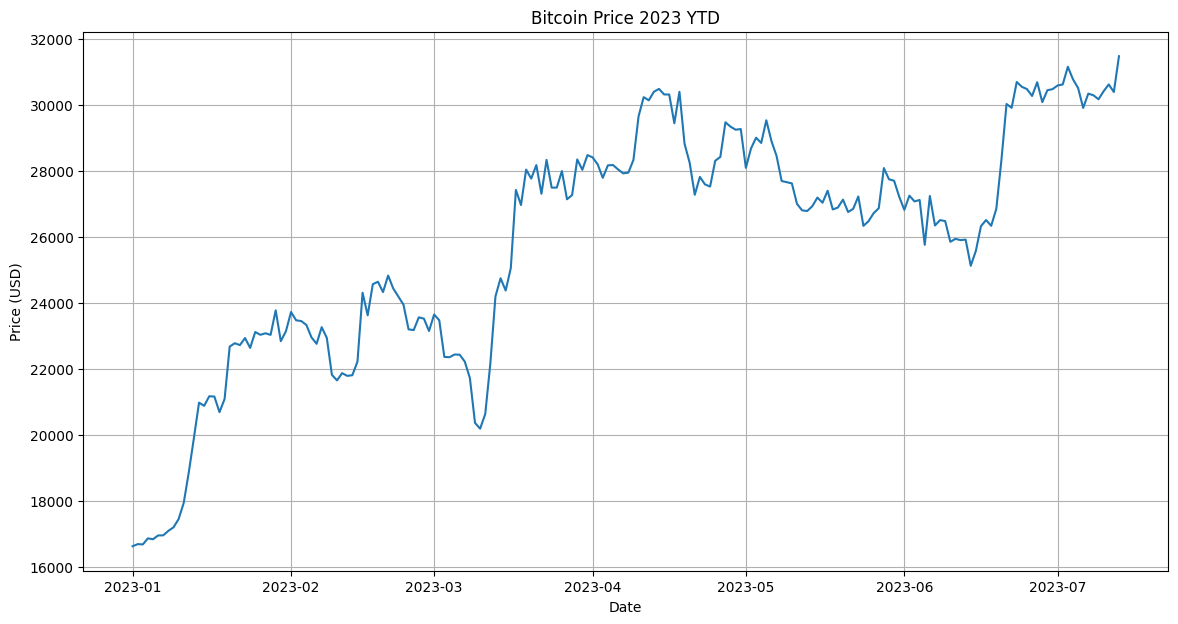 | ||
Bitcoin Chart Output |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,8 @@ | ||
# CodeInterpreterResponse | ||
The CodeInterpreterResponse contains the AI agent's response. | ||
|
||
It contains: | ||
|
||
- `content`: text response content | ||
- `files`: list of generated File attachments | ||
- `code_log`: log of executed code snippets |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,38 @@ | ||
# CodeInterpreterSession | ||
The CodeInterpreterSession is the main class that manages a conversational session between the user and AI agent. It handles starting, stopping and checking status of the secure isolated code execution environment. | ||
|
||
Key responsibilities: | ||
|
||
- Starting and stopping the compute session | ||
- Sending user input and files to the agent | ||
- Running code in the compute container | ||
- Retrieving output files and images | ||
- Managing the chat history | ||
- Logging | ||
|
||
It provides methods like: | ||
|
||
- `generate_response()`: Generate AI response for user input | ||
- `start() / stop()`: Start and stop the session | ||
- `log()`: Log messages | ||
- `show_code` - Callback to print code before running. | ||
|
||
|
||
The response generation happens through a pipeline: | ||
|
||
1. User input is parsed | ||
2. Code is executed if needed | ||
3. Files are processed if needed | ||
4. Final response is formatted and returned | ||
|
||
The `generate_response()` method handles this entire pipeline. | ||
|
||
Usage: | ||
|
||
```python | ||
from codeinterpreterapi import CodeInterpreterSession | ||
|
||
with CodeInterpreterSession() as session: | ||
response = session.generate_response("Plot a histogram of the data") | ||
print(response.content) # print AI response | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,24 @@ | ||
# CodeBox | ||
The CodeBox class provides the isolated secure environment for executing python code. It is used by the CodeInterpreterSession internally. | ||
|
||
It provides methods like: | ||
|
||
- `upload() / download()`: Upload and download files | ||
- `run()`: Run python code | ||
- `install()`: Install python packages | ||
|
||
The CodeBox handles setting up the environment, installing packages, running code, capturing output and making it available. | ||
|
||
It uses Docker containers under the hood to provide the isolated env. | ||
|
||
Usage: | ||
|
||
```python | ||
from codeboxapi import CodeBox | ||
|
||
codebox = CodeBox() | ||
codebox.upload("data.csv", b"1,2,3\ | ||
4,5,6") | ||
output = codebox.run("import pandas as pd; df = pd.read_csv('data.csv')") | ||
print(output.content) | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,8 @@ | ||
# Concepts Overview | ||
| name | description | | ||
|-|-| | ||
| CodeInterpreterSession | Main class that manages a code execution session | | ||
| CodeBox | Handles code execution in a sandboxed environment | | ||
| File | Represents a file for upload/download to CodeBox | | ||
| UserRequest | User input message with optional file attachments | | ||
| CodeInterpreterResponse | AI response message with optional files and code log | |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,64 @@ | ||
# Deployment | ||
CodeInterpreterAPI can be easily deployed to production using the CodeBox framework. | ||
|
||
## Prerequisites | ||
- CodeBox API key | ||
- Get your API key from [CodeBox](https://pay.codeboxapi.com/b/00g3e6dZX2fTg0gaEE) (get 70% with the code `BETA`) | ||
- CodeInterpreterAPI installed | ||
- `pip install codeinterpreterapi` | ||
|
||
## Setup | ||
|
||
Set the `CODEBOX_API_KEY` environment variable or directly in your code: | ||
|
||
```python | ||
from codeinterpreterapi import settings | ||
|
||
settings.CODEBOX_API_KEY = "sk-..." | ||
``` | ||
|
||
## Starting a Session | ||
|
||
To start a code interpreter session using CodeBox: | ||
|
||
```python | ||
from uuid import uuid4 | ||
from codeinterpreterapi import CodeInterpreterSession | ||
|
||
session_id = uuid4() | ||
|
||
session = CodeInterpreterSession.from_id(session_id) | ||
session.start() | ||
``` | ||
|
||
The `session_id` allows restoring the session later after restarting the application. | ||
|
||
## Generating Responses | ||
|
||
With the session started, you can now generate responses like normal: | ||
|
||
```python | ||
response = session.generate_response( | ||
"Plot a histogram of the iris dataset" | ||
) | ||
|
||
response.show() | ||
``` | ||
|
||
This will execute the code securely using CodeBox. | ||
|
||
## Stopping the Session | ||
|
||
Don't forget to stop the session when finished: | ||
|
||
```python | ||
session.stop() | ||
``` | ||
|
||
This will shutdown the CodeBox instance. | ||
|
||
## Next Steps | ||
|
||
- See the [CodeBox docs](https://codeboxapi.com/docs) for more details on deployment options. | ||
- Look at the [examples](https://github.com/shroominic/codebox-api/tree/main/examples) for more usage ideas. | ||
- Contact [Shroominic](https://twitter.com/shroominic) for assistance with scaling. |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,23 @@ | ||
# File | ||
The File class is used to represent files that are uploaded or downloaded during the session. | ||
|
||
It stores the filename and binary content of the file. | ||
|
||
It provides utility methods like: | ||
|
||
- `from_path()`: Create File from filesystem path | ||
- `from_url` - Create File from URL | ||
- `save()`: Save File to filesystem path | ||
- `show_image()`: Display image File | ||
|
||
Usage: | ||
|
||
```python | ||
from codeinterpreterapi import File | ||
|
||
file = File.from_path("image.png") | ||
file.show_image() # display image | ||
file.save("copy.png") # save copy | ||
``` | ||
|
||
File objects can be passed to `CodeInterpreterSession.generate_response` to make them available to the agent. |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,42 @@ | ||
# Installation | ||
## Install Dependencies | ||
|
||
To install, it's recommended to create a virtual environment (using `venv` in the example below): | ||
|
||
```bash | ||
python3 -m venv codeinterpreterenv | ||
source codeinterpreterenv/bin/activate | ||
``` | ||
|
||
Then install the package: | ||
|
||
```bash | ||
pip install "codeinterpreterapi[all]" | ||
``` | ||
|
||
Everything for local experiments are installed with the all extra. For deployments, you can use `pip install codeinterpreterapi` instead which does not install the additional dependencies. | ||
|
||
## Set Up Environment Variables | ||
|
||
|
||
You will also need to configure API keys for the AI model you want to use, either OpenAI, Anthropic, or Azure. | ||
|
||
For OpenAI, create a `.env` file with: | ||
|
||
```bash | ||
OPENAI_API_KEY=sk-... | ||
``` | ||
or export as an environment variable in your terminal: | ||
|
||
```bash | ||
export OPENAI_API_KEY=your_openai_api_key | ||
``` | ||
|
||
For Azure, use: | ||
|
||
```bash | ||
OPENAI_API_TYPE=azure | ||
OPENAI_API_VERSION=2023-07-01-preview | ||
OPENAI_API_BASE= | ||
DEPLOYMENT_NAME= | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,32 @@ | ||
# Analyzing the Iris Dataset | ||
```python | ||
from codeinterpreterapi import CodeInterpreterSession, File | ||
|
||
async def main(): | ||
# context manager for auto start/stop of the session | ||
async with CodeInterpreterSession() as session: | ||
# define the user request | ||
user_request = "Analyze this dataset and plot something interesting about it." | ||
files = [ | ||
File.from_path("examples/assets/iris.csv"), | ||
] | ||
|
||
# generate the response | ||
response = await session.generate_response( | ||
user_request, files=files | ||
) | ||
|
||
# output to the user | ||
print("AI: ", response.content) | ||
for file in response.files: | ||
file.show_image() | ||
|
||
|
||
if __name__ == "__main__": | ||
import asyncio | ||
|
||
asyncio.run(main()) | ||
``` | ||
|
||
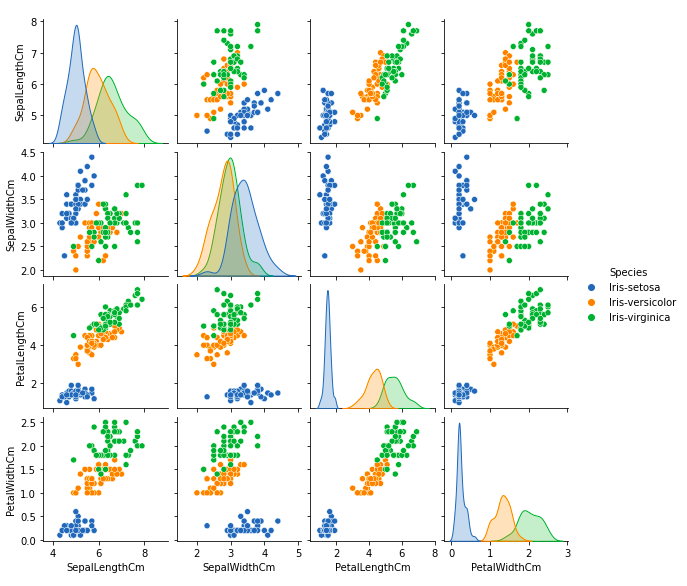 | ||
Iris Dataset Analysis Output |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,15 @@ | ||
# Using the Streamlit Webapp | ||
The streamlit webapp allows interacting with the API through a GUI: | ||
|
||
```bash | ||
streamlit run frontend/app.py --browser.gatherUsageStats=False | ||
``` | ||
|
||
This will launch the webapp where you can: | ||
|
||
- Write prompts and see results immediately | ||
- Upload files that get passed to the API | ||
- Download any files produced by the API | ||
- Switch between different models like GPT-3.5 Turbo | ||
|
||
So the webapp allows easily leveraging the API through a graphical interface. |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,19 @@ | ||
# Usage | ||
To create a session and generate a response: | ||
|
||
```python | ||
from codeinterpreterapi import CodeInterpreterSession, settings | ||
|
||
# set api key (or automatically loads from env vars) | ||
settings.OPENAI_API_KEY = "sk-***************" | ||
|
||
# create a session | ||
with CodeInterpreterSession() as session: | ||
# generate a response based on user input | ||
response = session.generate_response( | ||
"Plot the bitcoin chart of year 2023" | ||
) | ||
|
||
# output the response | ||
response.show() | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,18 @@ | ||
# UserRequest | ||
The UserRequest class represents the user input to the agent. | ||
|
||
It contains: | ||
|
||
- `content`: text content of user message | ||
- `files`: list of File attachments | ||
|
||
Usage: | ||
|
||
```python | ||
from codeinterpreterapi import UserRequest, File | ||
|
||
request = UserRequest( | ||
content="Here is an image", | ||
files=[File.from_path("image.png")] | ||
) | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,9 @@ | ||
# Welcome | ||
This project provides a LangChain implementation of the ChatGPT Code Interpreter. It allows you to have a back and forth chat with the AI assistant to get it to help with programming tasks, data analysis, and more. You can run everything local except the LLM using your own OpenAI API Key. | ||
|
||
Some key features: | ||
|
||
- Sandboxed execution of Python code snippets provided by the AI assistant using CodeBox. CodeBox is the simplest cloud infrastructure for your LLM Apps. | ||
- Automatic handling of file uploads/downloads | ||
- Support for stateful conversations with chat history | ||
- Extensible architecture to add custom tools and logic |