-
Notifications
You must be signed in to change notification settings - Fork 2.1k
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Media Filter RTD Provider : initial modul release (#10418)
* Added Media Filter Real Time Data Provider Module * fixed eslint errors * Modified MEDIAFILTER_EVENT_TYPE in unit tests * re-trigger pipeline * removed blank space * re-trigger pipelines * updated readme * added mediafilter to adloader.js and submodules.json
- Loading branch information
1 parent
683b5e6
commit 9c6cd73
Showing
5 changed files
with
281 additions
and
1 deletion.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,94 @@ | ||
/** | ||
* This module adds the Media Filter real-time ad monitoring and protection module. | ||
* | ||
* The {@link module:modules/realTimeData} module is required | ||
* | ||
* For more information, visit {@link https://www.themediatrust.com The Media Trust}. | ||
* | ||
* @author Mirnes Cajlakovic <[email protected]> | ||
* @module modules/mediafilterRtdProvider | ||
* @requires module:modules/realTimeData | ||
*/ | ||
|
||
import { submodule } from '../src/hook.js'; | ||
import { logError, generateUUID } from '../src/utils.js'; | ||
import { loadExternalScript } from '../src/adloader.js'; | ||
import * as events from '../src/events.js'; | ||
import CONSTANTS from '../src/constants.json'; | ||
|
||
/** @const {string} MEDIAFILTER_EVENT_TYPE - The event type for Media Filter. */ | ||
export const MEDIAFILTER_EVENT_TYPE = 'com.mediatrust.pbjs.'; | ||
/** @const {string} MEDIAFILTER_BASE_URL - The base URL for Media Filter scripts. */ | ||
export const MEDIAFILTER_BASE_URL = 'https://scripts.webcontentassessor.com/scripts/'; | ||
|
||
export const MediaFilter = { | ||
/** | ||
* Registers the Media Filter as a submodule of real-time data. | ||
*/ | ||
register: function() { | ||
submodule('realTimeData', { | ||
'name': 'mediafilter', | ||
'init': this.generateInitHandler() | ||
}); | ||
}, | ||
|
||
/** | ||
* Sets up the Media Filter by initializing event listeners and loading the external script. | ||
* @param {object} configuration - The configuration object. | ||
*/ | ||
setup: function(configuration) { | ||
this.setupEventListener(configuration.configurationHash); | ||
this.setupScript(configuration.configurationHash); | ||
}, | ||
|
||
/** | ||
* Sets up an event listener for Media Filter messages. | ||
* @param {string} configurationHash - The configuration hash. | ||
*/ | ||
setupEventListener: function(configurationHash) { | ||
window.addEventListener('message', this.generateEventHandler(configurationHash)); | ||
}, | ||
|
||
/** | ||
* Loads the Media Filter script based on the provided configuration hash. | ||
* @param {string} configurationHash - The configuration hash. | ||
*/ | ||
setupScript: function(configurationHash) { | ||
loadExternalScript(MEDIAFILTER_BASE_URL.concat(configurationHash), 'mediafilter', () => {}); | ||
}, | ||
|
||
/** | ||
* Generates an event handler for Media Filter messages. | ||
* @param {string} configurationHash - The configuration hash. | ||
* @returns {function} The generated event handler. | ||
*/ | ||
generateEventHandler: function(configurationHash) { | ||
return (windowEvent) => { | ||
if (windowEvent.data.type === MEDIAFILTER_EVENT_TYPE.concat('.', configurationHash)) { | ||
events.emit(CONSTANTS.EVENTS.BILLABLE_EVENT, { | ||
'billingId': generateUUID(), | ||
'configurationHash': configurationHash, | ||
'type': 'impression', | ||
'vendor': 'mediafilter', | ||
}); | ||
} | ||
}; | ||
}, | ||
|
||
/** | ||
* Generates an initialization handler for Media Filter. | ||
* @returns {function} The generated init handler. | ||
*/ | ||
generateInitHandler: function() { | ||
return (configuration) => { | ||
try { | ||
this.setup(configuration); | ||
} catch (error) { | ||
logError(`Error in initialization: ${error.message}`); | ||
} | ||
}; | ||
} | ||
}; | ||
|
||
// Register the module | ||
MediaFilter.register(); |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,37 @@ | ||
## Overview | ||
|
||
**Module:** The Media Filter | ||
**Type: **Real Time Data Module | ||
|
||
As malvertising, scams, and controversial and offensive ad content proliferate across the digital media ecosystem, publishers need advanced controls to both shield audiences from malware attacks and ensure quality site experience. With the market’s fastest and most comprehensive real-time ad quality tool, The Media Trust empowers publisher Ad/Revenue Operations teams to block a wide range of malware, high-risk ad platforms, heavy ads, ads with sensitive or objectionable content, and custom lists (e.g., competitors). Customizable replacement code calls for a new ad to ensure impressions are still monetized. | ||
|
||
[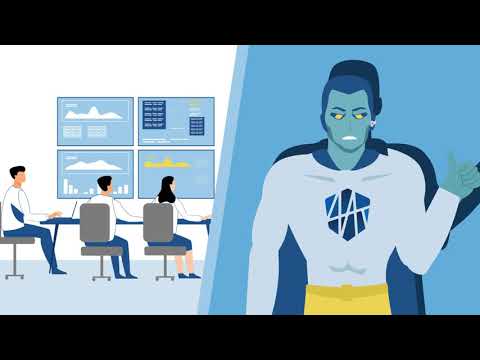](http://www.youtube.com/watch?v=VBHRiirge7s "Publishers' Ultimate Avenger: Media Filter") | ||
|
||
To start using this module, please contact [The Media Trust](https://mediatrust.com/how-we-help/media-filter/ "The Media Trust") to get a script and configuration hash for module configuration. | ||
|
||
## Integration | ||
|
||
1. Build Prebid bundle with The Media Filter module included. | ||
|
||
``` | ||
gulp build --modules=mediafilterRtdProvider | ||
``` | ||
|
||
2. Inlcude the bundled script in your application. | ||
|
||
## Configuration | ||
|
||
Add configuration entry to `realTimeData.dataProviders` for The Media Filter module. | ||
|
||
``` | ||
pbjs.setConfig({ | ||
realTimeData: { | ||
dataProviders: [{ | ||
name: 'mediafilter', | ||
params: { | ||
configurationHash: '<configurationHash>', | ||
} | ||
}] | ||
} | ||
}); | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
|
@@ -27,6 +27,7 @@ const _approvedLoadExternalJSList = [ | |
'clean.io', | ||
'a1Media', | ||
'geoedge', | ||
'mediafilter', | ||
'qortex' | ||
] | ||
|
||
|
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,147 @@ | ||
import * as utils from '../../../src/utils.js'; | ||
import * as hook from '../../../src/hook.js' | ||
import * as events from '../../../src/events.js'; | ||
import CONSTANTS from '../../../src/constants.json'; | ||
|
||
import { | ||
MediaFilter, | ||
MEDIAFILTER_EVENT_TYPE, | ||
MEDIAFILTER_BASE_URL | ||
} from '../../../modules/mediafilterRtdProvider.js'; | ||
|
||
describe('The Media Filter RTD module', function () { | ||
describe('register()', function() { | ||
let submoduleSpy, generateInitHandlerSpy; | ||
|
||
beforeEach(function () { | ||
submoduleSpy = sinon.spy(hook, 'submodule'); | ||
generateInitHandlerSpy = sinon.spy(MediaFilter, 'generateInitHandler'); | ||
}); | ||
|
||
afterEach(function () { | ||
submoduleSpy.restore(); | ||
generateInitHandlerSpy.restore(); | ||
}); | ||
|
||
it('should register and call the submodule function(s)', function () { | ||
MediaFilter.register(); | ||
|
||
expect(submoduleSpy.calledOnceWithExactly('realTimeData', sinon.match.object)).to.be.true; | ||
expect(submoduleSpy.called).to.be.true; | ||
expect(generateInitHandlerSpy.called).to.be.true; | ||
}); | ||
}); | ||
|
||
describe('setup()', function() { | ||
let setupEventListenerSpy, setupScriptSpy; | ||
|
||
beforeEach(function() { | ||
setupEventListenerSpy = sinon.spy(MediaFilter, 'setupEventListener'); | ||
setupScriptSpy = sinon.spy(MediaFilter, 'setupScript'); | ||
}); | ||
|
||
afterEach(function() { | ||
setupEventListenerSpy.restore(); | ||
setupScriptSpy.restore(); | ||
}); | ||
|
||
it('should call setupEventListener and setupScript function(s)', function() { | ||
MediaFilter.setup({ configurationHash: 'abc123' }); | ||
|
||
expect(setupEventListenerSpy.called).to.be.true; | ||
expect(setupScriptSpy.called).to.be.true; | ||
}); | ||
}); | ||
|
||
describe('setupEventListener()', function() { | ||
let setupEventListenerSpy, addEventListenerSpy; | ||
|
||
beforeEach(function() { | ||
setupEventListenerSpy = sinon.spy(MediaFilter, 'setupEventListener'); | ||
addEventListenerSpy = sinon.spy(window, 'addEventListener'); | ||
}); | ||
|
||
afterEach(function() { | ||
setupEventListenerSpy.restore(); | ||
addEventListenerSpy.restore(); | ||
}); | ||
|
||
it('should call addEventListener function(s)', function() { | ||
MediaFilter.setupEventListener(); | ||
expect(addEventListenerSpy.called).to.be.true; | ||
expect(addEventListenerSpy.calledWith('message', sinon.match.func)).to.be.true; | ||
}); | ||
}); | ||
|
||
describe('generateInitHandler()', function() { | ||
let generateInitHandlerSpy, setupMock, logErrorSpy; | ||
|
||
beforeEach(function() { | ||
generateInitHandlerSpy = sinon.spy(MediaFilter, 'generateInitHandler'); | ||
setupMock = sinon.stub(MediaFilter, 'setup').throws(new Error('Mocked error!')); | ||
logErrorSpy = sinon.spy(utils, 'logError'); | ||
}); | ||
|
||
afterEach(function() { | ||
generateInitHandlerSpy.restore(); | ||
setupMock.restore(); | ||
logErrorSpy.restore(); | ||
}); | ||
|
||
it('should handle errors in the catch block when setup throws an error', function() { | ||
const initHandler = MediaFilter.generateInitHandler(); | ||
initHandler({}); | ||
|
||
expect(logErrorSpy.calledWith('Error in initialization: Mocked error!')).to.be.true; | ||
}); | ||
}); | ||
|
||
describe('generateEventHandler()', function() { | ||
let generateEventHandlerSpy, eventsEmitSpy; | ||
|
||
beforeEach(function() { | ||
generateEventHandlerSpy = sinon.spy(MediaFilter, 'generateEventHandler'); | ||
eventsEmitSpy = sinon.spy(events, 'emit'); | ||
}); | ||
|
||
afterEach(function() { | ||
generateEventHandlerSpy.restore(); | ||
eventsEmitSpy.restore(); | ||
}); | ||
|
||
it('should emit a billable event when the event type matches', function() { | ||
const configurationHash = 'abc123'; | ||
const eventHandler = MediaFilter.generateEventHandler(configurationHash); | ||
|
||
const mockEvent = { | ||
data: { | ||
type: MEDIAFILTER_EVENT_TYPE.concat('.', configurationHash) | ||
} | ||
}; | ||
|
||
eventHandler(mockEvent); | ||
|
||
expect(eventsEmitSpy.calledWith(CONSTANTS.EVENTS.BILLABLE_EVENT, { | ||
'billingId': sinon.match.string, | ||
'configurationHash': configurationHash, | ||
'type': 'impression', | ||
'vendor': 'mediafilter', | ||
})).to.be.true; | ||
}); | ||
|
||
it('should not emit a billable event when the event type does not match', function() { | ||
const configurationHash = 'abc123'; | ||
const eventHandler = MediaFilter.generateEventHandler(configurationHash); | ||
|
||
const mockEvent = { | ||
data: { | ||
type: 'differentEventType' | ||
} | ||
}; | ||
|
||
eventHandler(mockEvent); | ||
|
||
expect(eventsEmitSpy.called).to.be.false; | ||
}); | ||
}); | ||
}); |