-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
This is the first version of spamwatch-go wrapper.
- Loading branch information
Showing
10 changed files
with
557 additions
and
0 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,64 @@ | ||
# <a href="https://telegram.me/SpamWatch"><img src="https://avatars.githubusercontent.com/u/37397813?s=200&v=4" width="35px" align="left"></img></a> SpamWatch API Go Wrapper | ||
|
||
[](https://pkg.go.dev/github.com/SpamWatch/spamwatch-go) [](http://perso.crans.org/besson/LICENSE.html) | ||
|
||
spamwatch-go is official Go wrapper for [SpamWatch API](https://api.spamwat.ch), which is fast, secure and requires no additional packages to be installed. | ||
|
||
<hr/> | ||
|
||
## Features | ||
|
||
- Can use custom SpamWatch API endpoint with the help of ClientOpts. | ||
- It's in pure go, no need to install any kind of plugin or include any kind of additional files. | ||
- No third party library bloat; only uses standard library. | ||
- Type safe; no weird `interface{}` logic. | ||
|
||
<hr/> | ||
|
||
## Getting started | ||
|
||
You can easily download the library with the standard `go get` command: | ||
|
||
```bash | ||
go get github.com/SpamWatch/spamwatch-go | ||
``` | ||
|
||
Full documentation of this API, can be found [here](https://docs.spamwat.ch/). | ||
|
||
<hr/> | ||
|
||
## Basic Usage | ||
|
||
```go | ||
package main | ||
|
||
import ( | ||
"fmt" | ||
|
||
"github.com/SpamWatch/spamwatch-go" | ||
) | ||
|
||
var client = spamwatch.Client("API_KEY", nil) | ||
|
||
func main() { | ||
ban, err := client.GetBan(777000) | ||
if err != nil { | ||
fmt.Println(err.Error()) | ||
return | ||
} | ||
fmt.Println(ban) | ||
} | ||
``` | ||
|
||
Still need more examples? Take a look at the [examples directory](examples). | ||
|
||
Ask your doubts at the [support group](https://telegram.me/SpamWatchSupport). | ||
|
||
<hr/> | ||
|
||
## License | ||
|
||
[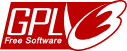](https://www.gnu.org/licenses/gpl-3.0.en.html#header) | ||
|
||
The spamwatch-go project is under the [GPL-3.0](https://opensource.org/licenses/GPL-3.0) license. You can find the license file [here](LICENSE). | ||
|
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,11 @@ | ||
package spamwatch | ||
|
||
const DEFAULT_API_URL = "https://api.spamwat.ch/" | ||
|
||
const SPAMWATCH_ERROR_PREFIX = "SpamWatch-Error" | ||
|
||
const UserPermission = "User" | ||
|
||
const AdminPermission = "Admin" | ||
|
||
const RootPermission = "Root" |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,32 @@ | ||
package spamwatch | ||
|
||
import ( | ||
"encoding/json" | ||
"fmt" | ||
"time" | ||
) | ||
|
||
func handleError(sw *SpamWatch, s int, b []byte, method string) error { | ||
if s != 200 && s != 201 && s != 204 { | ||
var e = Error{} | ||
err := json.Unmarshal(b, &e) | ||
if err != nil { | ||
return err | ||
} | ||
return &ErrorHandler{Err: &e, Spamwatch: sw, Method: method} | ||
} | ||
return nil | ||
} | ||
|
||
func (e *ErrorHandler) Error() string { | ||
switch e.Err.Code { | ||
case 401: | ||
return fmt.Sprintf("%s: Make sure your API Token is correct", SPAMWATCH_ERROR_PREFIX) | ||
case 403: | ||
return fmt.Sprintf("%s: Your token's permission '%s' is not high enough", SPAMWATCH_ERROR_PREFIX, e.Spamwatch.Token.Permission) | ||
case 429: | ||
return fmt.Sprintf("%s: Too Many Requests for method '%s': Try again in %d seconds", SPAMWATCH_ERROR_PREFIX, e.Method, e.Err.Until-time.Now().Unix()) | ||
default: | ||
return fmt.Sprintf("%s: %s: %d-%s", SPAMWATCH_ERROR_PREFIX, e.Method, e.Err.Code, e.Err.Description) | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,41 @@ | ||
package main | ||
|
||
import ( | ||
"fmt" | ||
|
||
"github.com/SpamWatch/spamwatch-go" | ||
) | ||
|
||
var client = spamwatch.Client("API_KEY", nil) | ||
|
||
func main() { | ||
// Getting a specific ban | ||
ban, err := client.GetBan(777000) | ||
if err != nil { | ||
fmt.Println(err.Error()) | ||
return | ||
} | ||
fmt.Println(ban) | ||
|
||
// Getting all bans | ||
bans, err := client.GetBans() | ||
if err != nil { | ||
fmt.Println(err.Error()) | ||
return | ||
} | ||
fmt.Println(bans) | ||
|
||
// Getting a list of banned ids | ||
bannedIds, err := client.GetBansMin() | ||
if err != nil { | ||
fmt.Println(err.Error()) | ||
return | ||
} | ||
fmt.Println(bannedIds) | ||
|
||
// Adding a ban | ||
client.AddBan(777000, "reason", "message") | ||
|
||
// Deleting a ban | ||
client.DeleteBan(777000) | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,27 @@ | ||
package main | ||
|
||
import ( | ||
"fmt" | ||
|
||
"github.com/SpamWatch/spamwatch-go" | ||
) | ||
|
||
var client = spamwatch.Client("API_KEY", nil) | ||
|
||
func main() { | ||
// Getting the API version | ||
v, err := client.Version() | ||
if err != nil { | ||
fmt.Println(err.Error()) | ||
return | ||
} | ||
fmt.Println(v) | ||
|
||
// Getting some stats | ||
stats, err := client.Stats() | ||
if err != nil { | ||
fmt.Println(err.Error()) | ||
return | ||
} | ||
fmt.Println(stats.TotalBansCount) | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,49 @@ | ||
package main | ||
|
||
import ( | ||
"fmt" | ||
|
||
"github.com/SpamWatch/spamwatch-go" | ||
) | ||
|
||
var client = spamwatch.Client("API_KEY", nil) | ||
|
||
func main() { | ||
// Getting your own Token | ||
myToken, err := client.GetSelf() | ||
if err != nil { | ||
fmt.Println(err) | ||
return | ||
} | ||
fmt.Println(myToken) | ||
|
||
// Getting all Tokens | ||
tokens, err := client.GetTokens() | ||
if err != nil { | ||
fmt.Println(err) | ||
return | ||
} | ||
fmt.Println(tokens) | ||
|
||
// Getting a specific Token | ||
Token, err := client.GetToken(1) | ||
if err != nil { | ||
fmt.Println(err) | ||
return | ||
} | ||
fmt.Println(Token) | ||
|
||
// Getting a Users tokens | ||
tokens, err = client.GetUserTokens(777000) | ||
if err != nil { | ||
fmt.Println(err) | ||
return | ||
} | ||
fmt.Println(tokens) | ||
|
||
// Creating a Token | ||
client.CreateToken(777000, spamwatch.UserPermission) | ||
|
||
// Retiring a specific Token | ||
client.DeleteToken(1) | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,3 @@ | ||
module github.com/SpamWatch/spamwatch-go | ||
|
||
go 1.17 |
Oops, something went wrong.